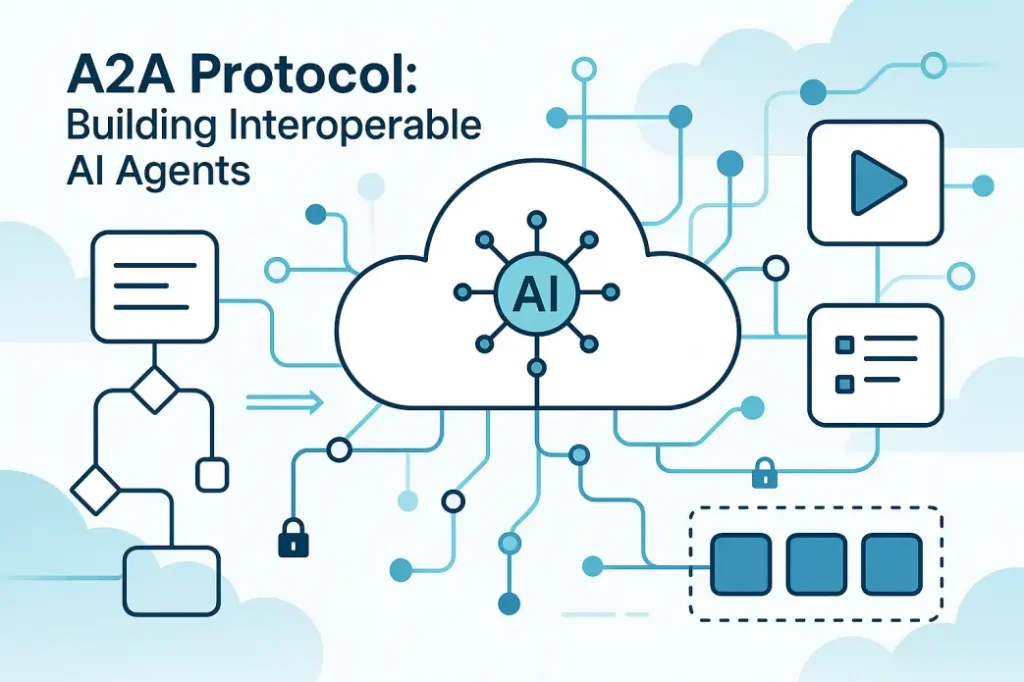
Introduction
The Agent-to-Agent (A2A) Protocol is an open-source framework introduced by Google to standardize communication between AI agents. It enables seamless data exchange and task collaboration across platforms, making it a powerful tool for developers building chatbots, automation tools, or enterprise AI systems. This guide focuses on what A2A is, how it works, and how developers can start using it with Python or JavaScript.
What is the A2A Protocol?
The A2A Protocol allows AI agents to communicate and collaborate using standardized web technologies. Key features include:
- HTTP-based APIs: Agents interact via RESTful APIs, leveraging familiar GET, POST, and other HTTP methods.
- JSON Data Format: Information is exchanged in JSON, ensuring compatibility across systems.
- Scalable Design: Supports interactions from simple chatbots to complex enterprise workflows.
A2A is beginner-friendly, requiring only basic knowledge of web technologies. Developers can use Python for backend logic or JavaScript for front-end interactions to build A2A-compatible agents.
Why A2A Matters for Developers
- Interoperability: Connects agents across platforms, breaking down silos.
- Accessibility: Built on standard APIs and JSON, it’s approachable for developers with web development experience.
- Open-Source: The A2A GitHub repository offers code samples and documentation.
- Future-Ready: Prepares developers for the growing demand for collaborative AI systems.
How A2A Works
A2A agents communicate via HTTP APIs, exchanging JSON payloads. For example, a chatbot agent might send a JSON request to a task automation agent to process data, receiving a response with the results. The protocol’s architecture supports both lightweight and complex interactions, scaling as needed.
Here’s a simplified flow:
- Agent A sends a POST request with JSON data to Agent B’s API endpoint.
- Agent B processes the request and responds with JSON.
- Both agents coordinate based on the exchanged data.
Getting Started with A2A
To start building A2A agents, follow these steps:
1. Learn the Core Technologies
A2A relies on web development fundamentals:
- Python or JavaScript: Python is ideal for backend agent logic, while JavaScript suits front-end or Node.js-based agents. For JavaScript, consider the book জাভাস্ক্রিপ্ট টিউটোরিয়াল-শিখুন গল্পে গল্পে সাথে 1100+ Exercise to build a strong foundation in Bangla.
- APIs and HTTP: Understand RESTful APIs and HTTP methods. Resources like FreeCodeCamp offer practical tutorials.
- JSON: Learn to structure and parse JSON data. Practice with tools like JSONLint.
For structured learning, consider a guided web development course like the one offered at Programming Hero, which covers JavaScript, APIs, and JSON.
2. Explore A2A Resources
- Google Developers Blog: Official A2A announcement and tutorials.
- A2A GitHub Repo: Sample code and documentation.
- A2A Official Site: Beginner-friendly protocol overview.
- Coursera: Courses on web development and AI fundamentals.
3. Build a Simple A2A Agent
Start with a basic project, such as a Python-based agent that communicates with another agent via A2A. Below is an example of a Python agent sending a JSON request:
import requests
import json
# Define the agent endpoint
agent_b_url = "https://agent-b.example.com/api"
# JSON payload
payload = {
"task": "process_data",
"data": {"value": 42}
}
# Send POST request to Agent B
response = requests.post(agent_b_url, json=payload)
# Parse response
if response.status_code == 200:
result = response.json()
print("Response from Agent B:", result)
else:
print("Error:", response.status_code)
Save this code, replace agent_b_url with a real endpoint, and test the interaction. The A2A GitHub repository provides additional examples.
4. Join the Community
- Hacker News: Discuss A2A and AI interoperability with developers.
- Agent2Agent Community Hub: Collaborate with A2A enthusiasts.
Common Misconceptions About A2A
- Myth: A2A is only for advanced developers.
- Fact: A2A is designed for beginners, requiring only basic Python or JavaScript skills.
- Myth: A2A needs complex infrastructure.
- Fact: It uses standard HTTP and JSON, suitable for small-scale projects.
- Myth: A2A is limited to chatbots.
- Fact: It supports diverse applications, from automation to enterprise AI.
Conclusion
The A2A Protocol empowers developers to build interoperable AI agents using familiar web technologies like Python, JavaScript, APIs, and JSON. By following this guide, you can start creating A2A agents with resources like the A2A GitHub repository and Google Developers Blog. Whether you’re enhancing chatbots or automating workflows, A2A opens new possibilities for innovation.